* 카카오맵 API 사용 가이드
2023.04.10 - [React] - [카카오맵 API] React TypeScript에서 카카오맵 불러오기
1. 커스텀 Hook 생성
첫 렌더링 시에 함수 실행을 막기 위한 Hook을 생성한다.
useEffect는 state가 변할 때 실행되는데 최초 렌더링 시에도 state가 설정되는 것을 확인 하고 실행된다. 이러한 상황을 막기 위한 Hook를 생성한다.
import { useEffect, useRef } from "react";
const useDidMountEffect = (func: any, deps: any) => {
const didMount = useRef(false);
useEffect(() => {
if (didMount.current) func();
else didMount.current = true;
}, deps);
};
export default useDidMountEffect;
- 최초 렌더링 시 kakao.maps이 load 안될 경우 실행을 막기 위한 커스텀 Hook 생성
2. Script 파라미터 추가
<script type="text/javascript" src="//dapi.kakao.com/v2/maps/sdk.js?appkey=발급받은 APP KEY를 넣으시면 됩니다.&autoload=false&libraries=services"></script>
- libraries=services : 주소 - 좌표 변환하는 객체 Geocoder를 사용하기 위해서 필요하다.
3. 코드 작성
declare global {
interface Window {
kakao: any;
}
}
export default function KakaoMap() {
const [map, setMap] = useState<any>();
const [marker, setMarker] = useState<any>();
// 1) 카카오맵 불러오기
useEffect(() => {
window.kakao.maps.load(() => {
const container = document.getElementById("map");
const options = {
center: new window.kakao.maps.LatLng(33.450701, 126.570667),
level: 3,
};
setMap(new window.kakao.maps.Map(container, options));
setMarker(new window.kakao.maps.Marker());
});
}, []);
// 2) 최초 렌더링 시에는 제외하고 map이 변경되면 실행
useDidMountEffect(() => {
window.kakao.maps.event.addListener(
map,
"click",
function (mouseEvent: any) {
// 주소-좌표 변환 객체를 생성합니다
var geocoder = new window.kakao.maps.services.Geocoder();
geocoder.coord2Address(
mouseEvent.latLng.getLng(),
mouseEvent.latLng.getLat(),
(result: any, status: any) => {
if (status === window.kakao.maps.services.Status.OK) {
var addr = !!result[0].road_address
? result[0].road_address.address_name
: result[0].address.address_name;
// 클릭한 위치 주소를 가져온다.
console.log(addr)
// 기존 마커를 제거하고 새로운 마커를 넣는다.
marker.setMap(null);
// 마커를 클릭한 위치에 표시합니다
marker.setPosition(mouseEvent.latLng);
marker.setMap(map);
}
}
);
}
);
}, [map]);
return(
<div>
<div id="map" style={{ width: "100%", height: "400px" }}></div>
<div>
);
}
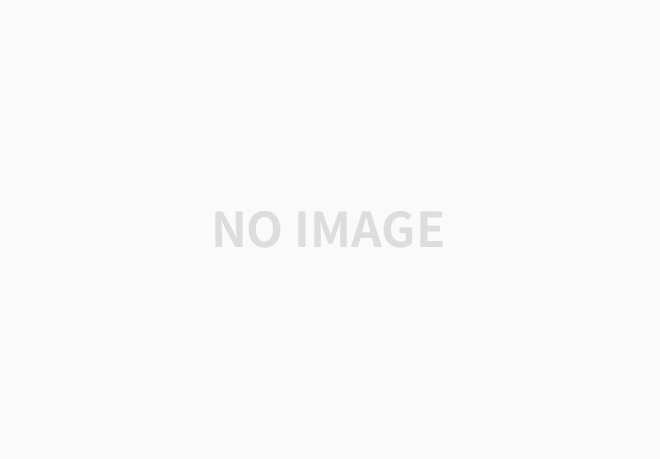
반응형
'React' 카테고리의 다른 글
[React] TypeScript에서 텍스트 편집기(React-Quill) 사용 방법 (0) | 2023.04.20 |
---|---|
[카카오맵 API / 카카오 주소 API] 검색된 주소를 카카오 맵에 표시하는 방법 (0) | 2023.04.18 |
[카카오맵 API] React TypeScript에서 Geolocation를 사용한 현재 위치 표시 방법 (0) | 2023.04.11 |
[카카오맵 API] React TypeScript에서 카카오맵 불러오기 (0) | 2023.04.10 |
[카카오 주소 API] TypeScript에서 카카오(다음) 주소 API 사용 방법 (0) | 2023.03.29 |